React is a popular JavaScript library used for building dynamic user interfaces. One of the most important features of React is its ability to manage state using hooks, specifically the useState hook. In this article, we will discuss the use of useState in React and how it can be used to manage state in your application.
useState is a built-in hook in React that allows you to add state to your functional components. It is used to manage the state of a component and update it based on user interactions or other events. With useState, you can easily create and update state variables within a functional component without having to use class-based components.
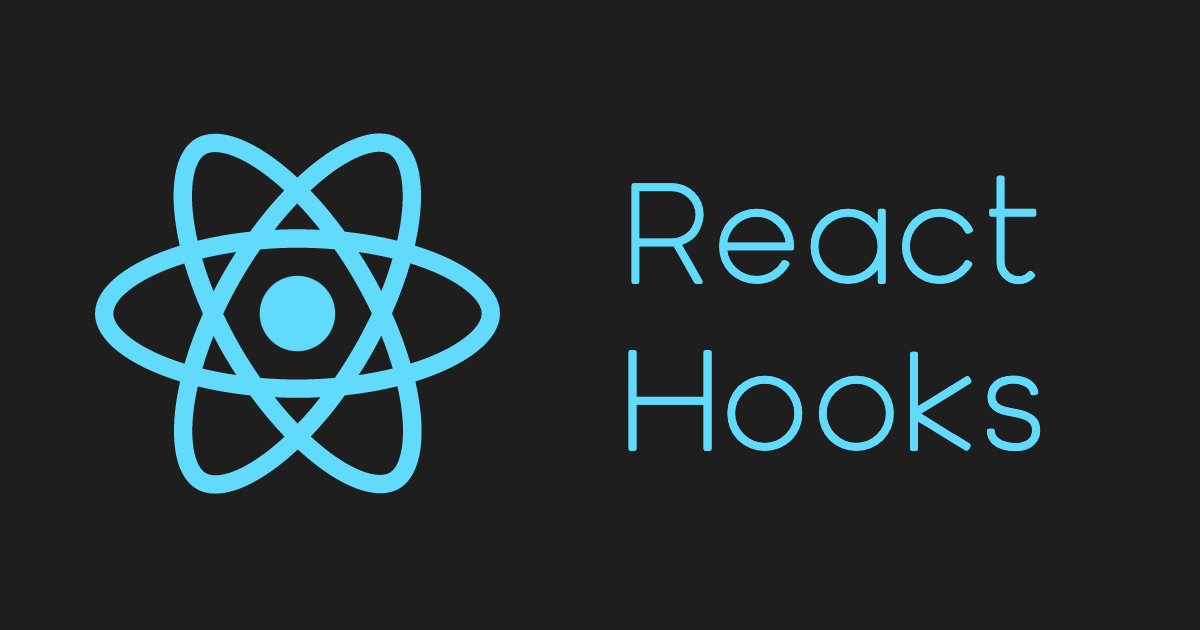
Benefits of Using React useState Hook:
The useState hook provides several benefits for managing state in your React application:
- Easy to Use: The useState hook is very easy to use and understand. It simplifies the process of managing state in your functional components.
- Lightweight: Since useState is a built-in hook in React, it is very lightweight and doesn't require any additional libraries or dependencies.
- Simple Syntax: The syntax for using the useState hook is very straightforward. You don't have to worry about complicated class-based components and lifecycle methods.
- Scalable: The useState hook is scalable and can be used for managing state in small and large applications.
Advanced Techniques with useState Hook:
There are several advanced techniques that you can use with the useState hook to manage state in your React application. Here are a few examples:
1. Using Object for State: You can use an object to manage multiple pieces of state in a single useState hook. Here is an example:
const [state, setState] = useState({ count: 0, name: 'John' });
2. Using Array for State: You can also use an array to manage multiple pieces of state in a single useState hook. Here is an example:
const [items, setItems] = useState([]);
3. Functional Updates: You can also use functional updates with useState to update state based on the previous state value. Here is an example:
setCount(prevCount => prevCount + 1);
Best Practices for Using React useState Hook:
While using the useState hook in React can simplify state management in functional components, it's important to follow some best practices to ensure that your code is efficient, maintainable, and scalable.
1. Keep State Variables Simple and Focused: When using useState, it's important to keep the state variables simple and focused. Avoid adding multiple state variables that are closely related or that share common properties.
For example, if you are building a form component, it might be tempting to create separate state variables for each input field. However, this can quickly become unwieldy as the number of fields grows. Instead, consider using a single state variable to store all of the form data.
2. Use Immutability to Update State Variables: When updating state variables with the useState hook, it's important to use immutability to ensure that the component is re-rendered when the state changes. Avoid directly modifying state variables, as this can lead to unpredictable behavior.
Instead, use the spread operator or Object.assign() to create a new copy of the state variable with the updated values.
3. Minimize the Number of Render Calls: When using useState, it's important to minimize the number of render calls to improve the performance of your application. This can be achieved by using memoization or shouldComponentUpdate to prevent unnecessary re-renders.
For example, if your component has a complex data structure, you might use the useMemo hook to memorize the data and only recalculate it when the dependencies change.
4. Use useEffect to Handle Side Effects: When using useState to manage state, it's common to use the useEffect hook to handle side effects, such as fetching data from an API or updating the DOM.
To ensure that your code is maintainable and scalable, it's important to separate the logic for managing state and handling side effects. Avoid adding complex logic to the useState hook, as this can make the code harder to read and maintain.
5. Use Custom Hooks to Share Stateful Logic: When you find yourself using the same state variables and logic in multiple components, it might be time to create a custom hook to share that stateful logic.
Custom hooks can simplify the process of reusing stateful logic and improve the maintainability and scalability of your code. For example, you might create a useFetch hook to handle API requests in multiple components.
Following these best practices can help you to use the useState hook effectively in your React applications. By keeping state variables simple and focused, using immutability to update state variables, minimizing render calls, using useEffect to handle side effects, and using custom hooks to share stateful logic, you can create maintainable and scalable React applications with ease.
Common Mistakes to Avoid When Using React useState Hook:
Here are some common mistakes to avoid when using the useState hook in your React application:
1. Not Initializing State: Always initialize your state variables with a default value, otherwise you will get errors when trying to update them.
2. Changing State Directly: Do not change the state variable directly, always use the setState function to update it.
3. Storing Computed Values in State: Avoid storing computed values in state, as this can lead to unnecessary re-renders and performance issues.
4. Using useState in Class Components: The useState hook is only meant to be used in functional components. If you need to manage state in a class component, use the setState method instead.
Conclusion
The useState hook is a powerful tool for managing state in your React applications. It simplifies the process of adding state to your functional components and provides several benefits, such as lightweight syntax and easy scalability. By following best practices and avoiding common mistakes, you can use the useState hook to build efficient and maintainable React applications.
For more information check out this React Usestate blog from CopyCat.